android-wheel having good example of time picker like iphone
how used android-wheel like Timepickerdialog ?
Follow below steps you can get output result like this dialog box..
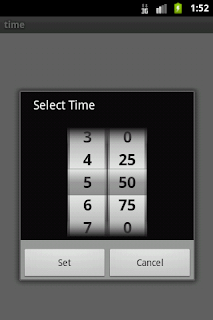
Step 1. take checkout source code of android-wheel library project from this link http://android-wheel.googlecode.com/svn/trunk/wheel
Step 2. import project inside eclipse as android project
Step 3. Add this project as a library in your android project
Step 4. create a layout.xml for timepicker (example name of file :- wheeltime.xml)
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="horizontal" android:gravity="center" > <kankan.wheel.widget.WheelView android:id="@+id/hours" android:layout_width="60sp" android:layout_height="wrap_content" android:paddingRight="5sp" /> <kankan.wheel.widget.WheelView android:id="@+id/minutes" android:layout_width="60sp" android:layout_height="wrap_content" android:paddingLeft="5sp" /> </LinearLayout>
Step 5. call function that create android.app.Dialog with this layout. see below code
private void Timepick(Context cons, int curHours, int curMinutes) { LayoutInflater inf = LayoutInflater.from(cons); //layout of alert box contains View view = inf.inflate(R.layout.wheeltime, null); //hours wheel final WheelView hours = (WheelView) view.findViewById(R.id.hours); hours.setViewAdapter(new NumericWheelAdapter(cons, 0, 23)); hours.setCyclic(true); //minute wheel final WheelView mins = (WheelView) view.findViewById(R.id.minutes); mins.setViewAdapter(new NumericWheelAdapter(cons, 0, 59)); mins.setCyclic(true); new AlertDialog.Builder(cons) .setMessage("Select Time") .setView(view) .setPositiveButton("Set", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int which) { // TODO Auto-generated method stub //write code of lines, whatever want to with value of this timepicker //hour value= hours.getCurrentItem(); //minute value = mins.getCurrentItem(); } }) .setNegativeButton("Cancel", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int which) { // TODO Auto-generated method stub return; } }).create().show(); // set time hours.setCurrentItem(curHours); mins.setCurrentItem(curMinutes); }